本文最后更新于 2024年8月23日 下午
0、简介
Selenium 可以在没有人参与的情况下使用浏览器,并通过代码实现自动化流程,例如模拟用户输入、提交表单。
1、安装
- 首先安装selenium库
查看浏览器版本
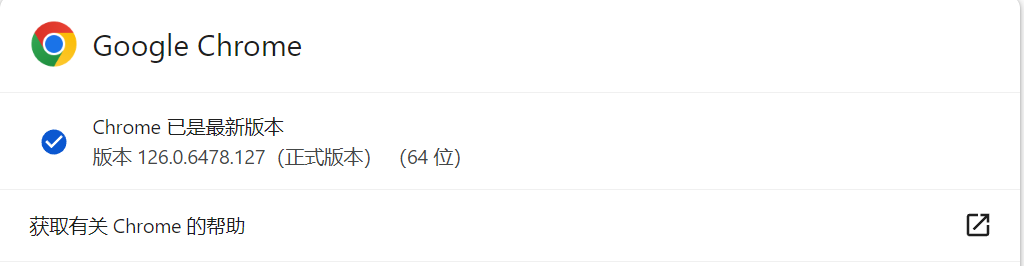
3.下载浏览器驱动文件
Chrome驱动下载地址:https://googlechromelabs.github.io/chrome-for-testing/
Firefox驱动下载地址:https://github.com/mozilla/geckodriver/releases
下载对应版本的驱动文件,把文件放到Python根目录。
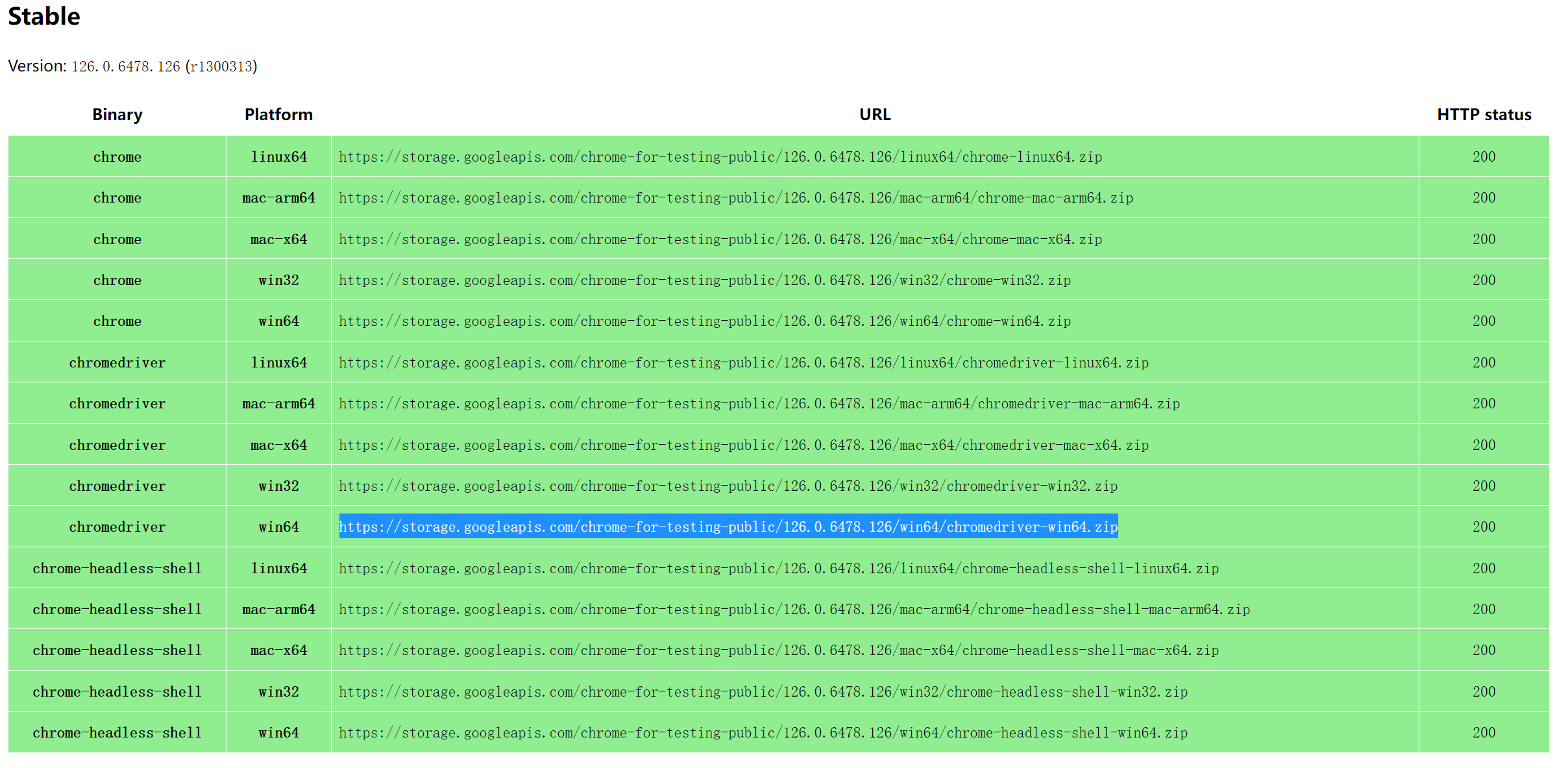
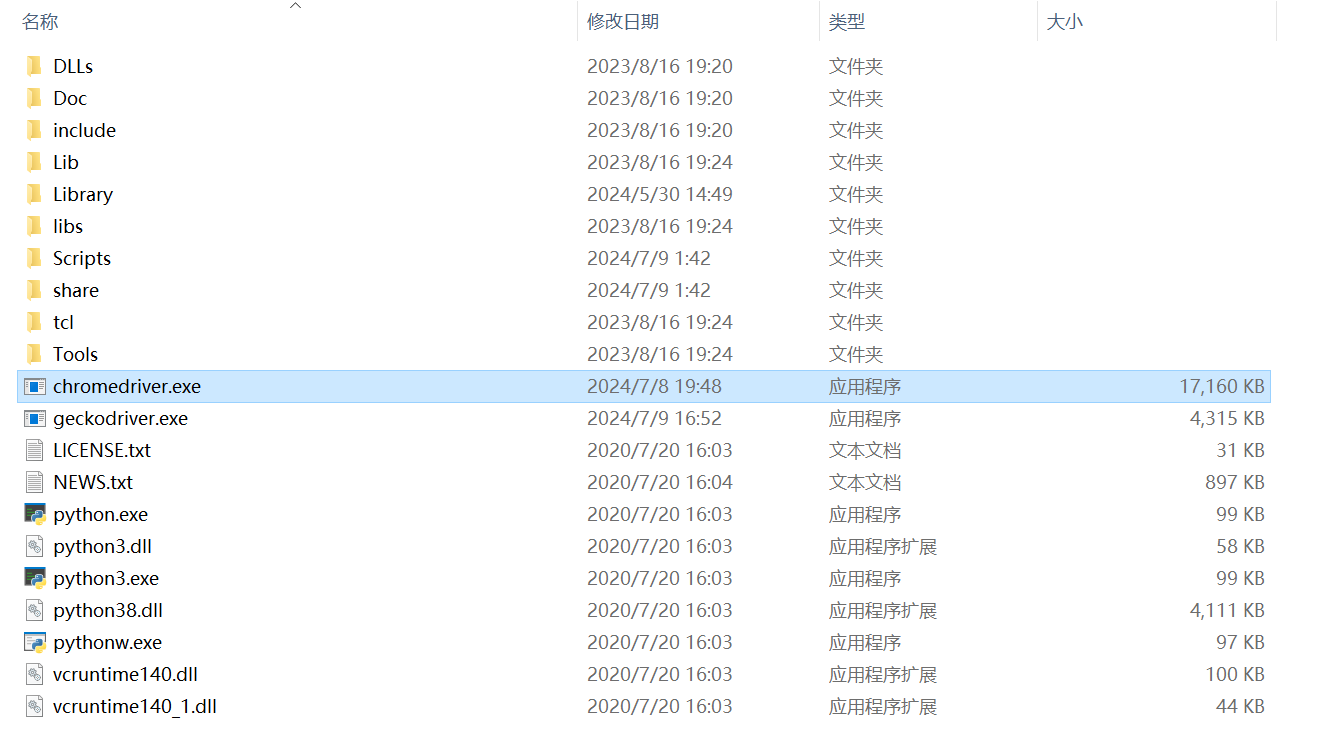
2、演示代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| import time from selenium import webdriver from selenium.webdriver.common.by import By
input_location = (By.XPATH, '//*[@id="ww"]') button_location = (By.XPATH, '//*[@id="s_btn_wr"]') news_location = (By.XPATH, '//*[@id="1"]/div/h3/a')
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--incognito")
chrome_options.add_experimental_option("excludeSwitches", ["enable-automation", "enable-logging"])
browser = webdriver.Chrome(chrome_options)
browser.get("https://news.baidu.com/")
browser.maximize_window()
browser.implicitly_wait(5)
element = browser.find_element(*input_location).send_keys("熊猫")
element = browser.find_element(*button_location).click()
text = browser.find_element(*news_location).text print(text)
time.sleep(3)
browser.quit()
|
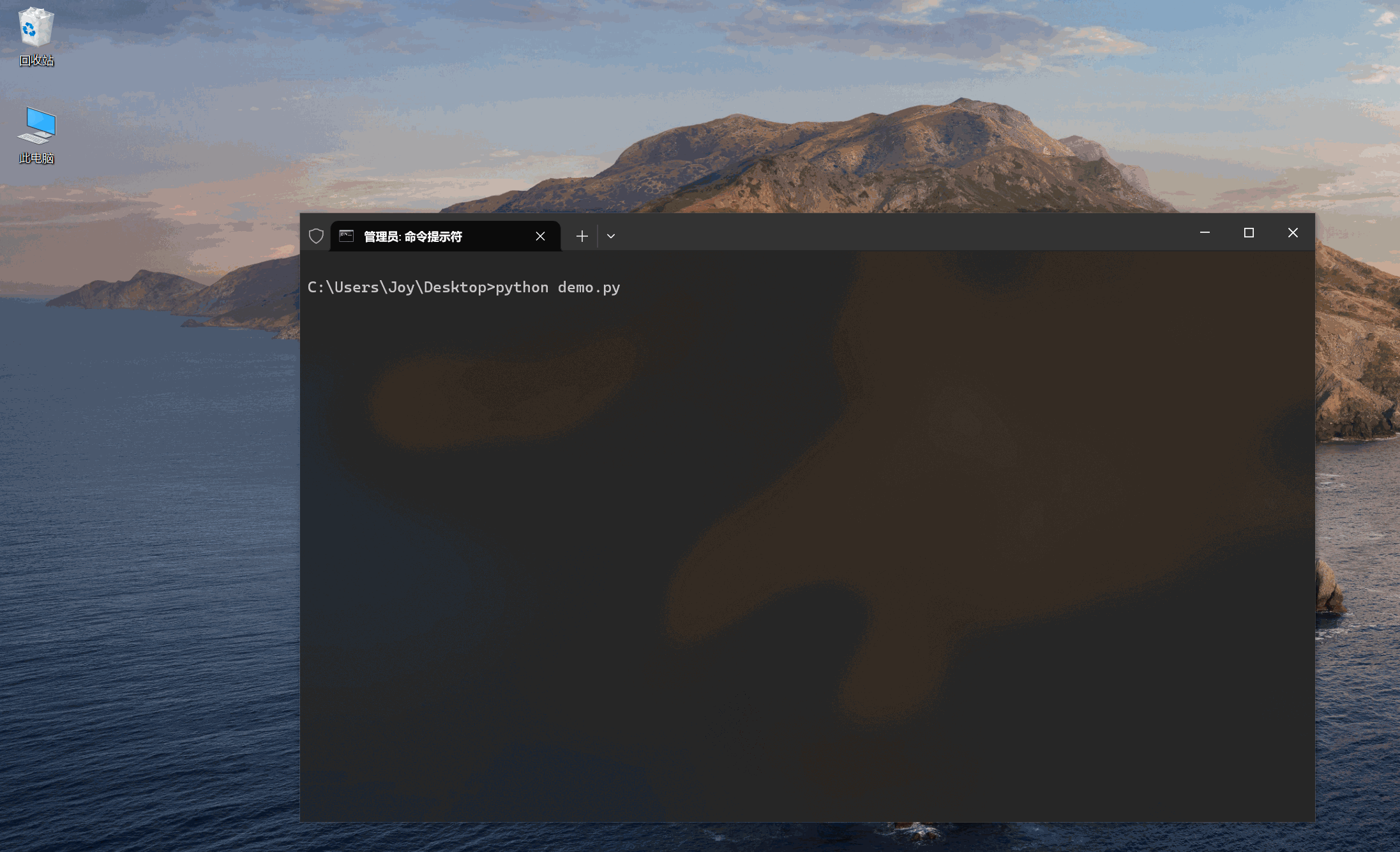
3、扩展
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| """ 初始化 Chrome 浏览器。 """ chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--incognito")
chrome_options.add_argument("--disable-cach")
chrome_options.add_argument("--disable-plugins")
chrome_options.add_argument("--disable-notifications")
chrome_options.add_argument("--disable-component-update")
chrome_options.add_argument("--ignore-certificate-errors")
chrome_options.add_experimental_option("useAutomationExtension", False)
chrome_options.add_experimental_option("excludeSwitches", ["enable-automation", "enable-logging"])
browser = webdriver.Chrome(chrome_options)
browser.maximize_window()
browser.implicitly_wait(5)
""" 初始化 Firefox 浏览器。 """ firefox_options = webdriver.FirefoxOptions()
firefox_options.set_preference("app.update.auto", False) firefox_options.set_preference("app.update.enabled", False)
firefox_options.set_preference("browser.ssl_override_behavior", 1)
firefox_options.set_preference("dom.webnotifications.enabled", False)
firefox_options.set_preference("browser.dom.window.dump.enabled", False)
firefox_options.set_preference("browser.console.showInPanel", False)
firefox_options.set_preference("toolkit.telemetry.reportingpolicy.firstRun", False)
browser = webdriver.Firefox(firefox_options)
browser.maximize_window()
browser.implicitly_wait(5)
|